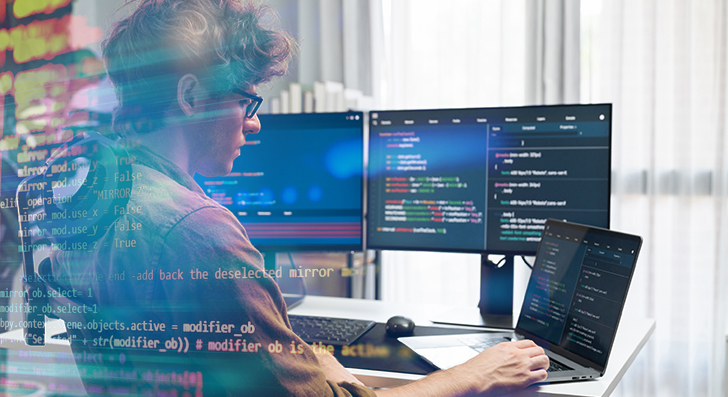
Scalability usually means your application can deal with growth—extra end users, a lot more data, and more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of the plan from the start. Many apps are unsuccessful whenever they grow rapidly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early about how your procedure will behave under pressure.
Start off by designing your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These patterns split your application into smaller, independent areas. Each individual module or services can scale on its own devoid of influencing the whole method.
Also, contemplate your databases from working day 1. Will it want to manage one million buyers or just a hundred? Pick the correct sort—relational or NoSQL—determined by how your facts will mature. Strategy for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present-day disorders. Think about what would happen In case your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that aid scaling, like information queues or celebration-pushed systems. These help your application cope with additional requests devoid of finding overloaded.
If you Create with scalability in mind, you are not just planning for achievement—you are lowering long term headaches. A perfectly-prepared process is simpler to maintain, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the Right Databases
Picking out the suitable databases is actually a crucial Section of making scalable apps. Not all databases are developed exactly the same, and using the Completely wrong you can slow you down or even cause failures as your application grows.
Get started by comprehension your info. Is it remarkably structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are powerful with interactions, transactions, and consistency. In addition they help scaling techniques like examine replicas, indexing, and partitioning to deal with additional site visitors and data.
When your info is a lot more flexible—like person activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured information and might scale horizontally more simply.
Also, take into consideration your go through and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of significant write throughput, and even function-centered knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need Sophisticated scaling characteristics now, but picking a databases that supports them means you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain styles. And often keep an eye on database functionality while you increase.
In a nutshell, the best databases is dependent upon your app’s construction, speed requirements, And just how you assume it to increase. Get time to pick wisely—it’ll save a lot of trouble afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your application grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular will work. Maintain your functions small, targeted, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to run or works by using a lot of memory.
Next, have a look at your database queries. These generally slow points down greater than the code alone. Make certain Each individual query only asks for the data you really need. Prevent Choose *, which fetches all the things, and as an alternative select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular across substantial tables.
In the event you observe the same info remaining requested over and over, use caching. Retail store the outcomes briefly applying tools like Redis or Memcached and that means you don’t need to repeat high-priced functions.
Also, batch your databases operations whenever you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with substantial datasets. Code and queries that work good with one hundred information may possibly crash every time they have to handle 1 million.
In brief, scalable apps are quickly applications. Maintain your code restricted, your queries lean, and use caching when needed. These actions aid your application remain clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more users and much more site visitors. If anything goes as a result of one server, it'll rapidly become a bottleneck. That’s exactly where load balancing and caching come in. These two tools help keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic throughout many servers. In place of just one server undertaking every one of the perform, the load balancer routes customers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused immediately. When end users request the same facts once again—like an item website page or maybe a profile—you don’t must fetch it from the databases each and every time. You can provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near the user.
Caching lowers database load, Gustavo Woltmann news enhances velocity, and helps make your application a lot more economical.
Use caching for things that don’t transform typically. And always be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application handle a lot more buyers, stay rapidly, and recover from troubles. If you propose to grow, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that allow your application improve easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, minimize set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to purchase hardware or guess potential capability. When targeted traffic boosts, you could increase a lot more assets with only a few clicks or routinely employing car-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app rather than handling infrastructure.
Containers are One more crucial Instrument. A container packages your application and all the things it really should operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your app concerning environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Software for this.
Once your app uses various containers, equipment like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your app crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments implies you could scale quickly, deploy easily, and Recuperate quickly when troubles happen. If you need your application to expand without the need of limitations, get started working with these tools early. They preserve time, cut down threat, and assist you stay focused on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when items go Erroneous. Checking helps you see how your app is undertaking, location troubles early, and make superior decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app way too. Control just how long it's going to take for buyers to load pages, how often errors occur, and exactly where they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a company goes down, it is best to get notified immediately. This helps you take care of challenges rapid, generally ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it triggers real destruction.
As your app grows, visitors and details maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about understanding your process and making sure it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for huge organizations. Even compact apps will need a strong Basis. By building meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly with no breaking stressed. Begin modest, Imagine large, and Make smart.